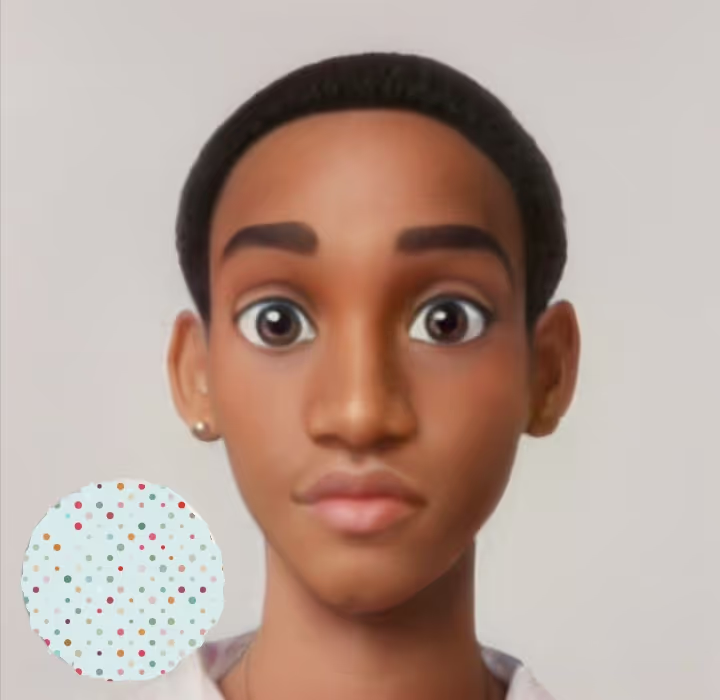
Golang Append (A Complete Guide)
What is the append method in Golang?
The append method is a built-in method in Golang used to add more elements to a the end of a slice. It is a variadic function that takes a slice and one or more elements to append to the slice. The append method returns a new slice with the new elements appended to the end of the slice.
How to use the append method in Golang
To use the append method in Golang, we must pass the slice and the elements to append to the slice as arguments to the append method as shown below.
package main
import "fmt"
func main() {
slice := []int{1, 2, 3}
slice = append(slice, 4, 5, 6)
fmt.Println(slice)
}
The above code will return a new slice with the new elements appended to the end of the slice.
[1 2 3 4 5 6]
How to append a slice to another slice in Golang
To append a slice to another slice in Golang, we must pass the slice and the slice to append to the slice as arguments with the ellipsis operator (…). The ellipsis operator is used to unpack the slice and pass the elements of the slice as arguments to the append method.
...
s1 := []int{1, 2, 3}
s2 := []int{4, 5, 6}
s1 = append(s1, s2...)
fmt.Println(s1)
The output would be:
[1 2 3 4 5 6]
How to append a slice to a map in Golang
To append a slice to a map in Golang, we must pass the map and the slice to append to the map as arguments with the ellipsis operator (…).
...
m := map[string][]int{"a": {1, 2, 3}}
s := []int{4, 5, 6}
m["a"] = append(m["a"], s...)
fmt.Println(m)
The output would be:
map[a:[1 2 3 4 5 6]]
How to append a slice to a struct in Golang
To append a slice to a struct in Golang, we must pass the struct and the slice to append to the struct as arguments with the ellipsis operator (…).
...
type Person struct {
Name string
Age int
Hobbies []string
}
p := Person{
Name: "John",
Age: 30,
Hobbies: []string{"Reading", "Writing"},
}
hobbies := []string{"Coding", "Gaming"}
p.Hobbies = append(p.Hobbies, hobbies...)
fmt.Println(p)
The output would be:
{John 30 [Reading Writing Coding Gaming]}
How to delete an element from a slice in Golang
To delete an element from a slice in Golang, we must pass a slice and the index of the element to delete as arguments to the append method. The append method will return a new slice with the element at the given index deleted.
...
slice := []int{1, 2, 3, 4, 5}
slice = append(slice[:2], slice[3:]...)
fmt.Println(slice)
The output would be:
[1 2 4 5]
In the above code, we are deleting the element at index 2 from the slice, so we are passing the slice from index 0 to index 2 and the slice from index 3 to the end of the slice as arguments to the append method. Since starting index is inclusive and the ending index is exclusive, the element at index 2 will be deleted.
How to append to a file in Golang
To append to a file in Golang, we must open the file in append mode using os.OpenFile
method. Then we must pass the file and the data to append to the file as arguments to the Write
method.
...
file, err := os.OpenFile("file.txt", os.O_APPEND|os.O_WRONLY, 0644)
if err != nil {
panic(err)
}
defer file.Close()
if _, err := file.Write([]byte("Hello World")); err != nil {
panic(err)
}
In the above code, we are opening file.txt
in append mode and writing Hello World
to the file.
How to append a string to a slice in Golang
To append a string to a slice in Golang, we must pass the slice and the string to append to the slice as arguments to the append
method.
...
slice := []string{"a", "b", "c"}
slice = append(slice, "d")
fmt.Println(slice)
The output would be:
[a b c d]
How to append a map to another map in Golang
To append a map to another map in Golang we must use the reflect
package. The reflect
package provides utilities for reflection, allowing a program to manipulate objects with arbitrary types. The reflect
package is used to get the value of a map and append it to another map.
...
m1 := map[string]int{"a": 1, "b": 2}
m2 := map[string]int{"c": 3, "d": 4}
for k, v := range m2 {
reflect.ValueOf(m1).SetMapIndex(reflect.ValueOf(k), reflect.ValueOf(v))
}
fmt.Println(m1)
The output would be:
map[a:1 b:2 c:3 d:4]
In the above code, we are using the reflect
package to get the map m2
value and append it to the map m1
. The reflect
package provides the ValueOf
method to get the value of a map. The SetMapIndex
method is used to append the value of the map to another map.
How to append an array to another array in Golang
Appending to an array is not possible in Golang because array size is fixed. To append an array to another array in Golang, we must convert the array to a slice and then append the slice to another slice.
...
a1 := [3]int{1, 2, 3}
a2 := [3]int{4, 5, 6}
s1 := a1[:]
s2 := a2[:]
s1 = append(s1, s2...)
fmt.Println(s1)
The output would be:
[1 2 3 4 5 6]
In the above code, we are converting the array a1
and a2
to slices and then appending the slices to another slice.
How to append multiple slices to a slice in Golang
To append multiple slices to a slice in Golang, we must pass the slice and the slices to append to the slice as arguments with the ellipsis operator (…).
...
s1 := []int{1, 2, 3}
s2 := []int{4, 5, 6}
s3 := []int{7, 8, 9}
s1 = append(s1, s2...)
s1 = append(s1, s3...)
fmt.Println(s1)
The output would be:
[1 2 3 4 5 6 7 8 9]
How to append to a nil slice in Golang
To append to a nil slice in Golang, we must pass the nil slice and the elements to append to the slice as arguments to the append
method.
...
var slice []int
slice = append(slice, 1, 2, 3)
fmt.Println(slice)
This is like creating a new slice and appending the elements to the new slice.
The output would be:
[1 2 3]
How to append to the front of a slice in Golang
To append to the front of a slice in Golang, we need to pass first the element to append to the slice and then the slice as arguments to the append
method. This is possible since a new slice is created when we append it to a slice.
...
slice := []int{1, 2, 3}
slice = append([]int{0}, slice...)
fmt.Println(slice)
The output would be:
[0 1 2 3]
The same would apply to append a slice to the front of another slice.
How to append bytes to a slice in Golang
To append bytes to a slice in Golang, we must pass the slice and the bytes to append to the slice as arguments to the append
method.
...
slice := []byte{1, 2, 3}
slice = append(slice, 4)
fmt.Println(slice)
The output would be:
[1 2 3 4]
Tl;dr
Append method is a key method in Golang as it gives us the ability to add elements to a slice in various ways and of various types.