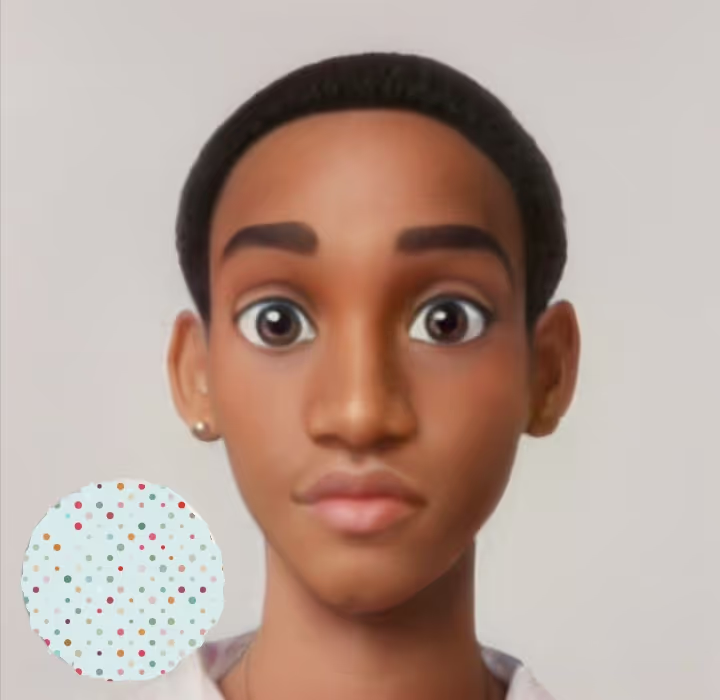
Golang Assert
What is Assert in Golang?
Assert is a package that provides a set of helper functions that make it easier to test various properties in your Go programs. It is intended to be used in the xtest files of your package, as an aid in writing tests.
How to use Assert in Golang
Installation
go get github.com/stretchr/testify/assert
Example
package main
import (
"fmt"
)
func greeting() string {
return "Hello, World!"
}
func main() {
fmt.Println(greeting())
}
Test file
package main
import (
"testing"
"github.com/stretchr/testify/assert"
)
func TestGreeting(t *testing.T) {
assert.Equal(t, "Hello, World!", greeting())
}
Output:
go test -v
=== RUN TestGreeting
--- PASS: TestGreeting (0.00s)
PASS
ok assert 0.010s
Major Assert Methods
1. Equal and NotEqual
assert.Equal()
and assert.NotEqual()
are used to check if the two objects are equal or not. It takes three arguments, the first one is the testing object, the second one is the expected object and the third one is the actual object.
...
assert.Equal(t, "Hello, World!", greeting())
assert.NotEqual(t, "Hello, World!", "Hello, Universe!")
3. Nil and NotNil
assert.Nil()
and assert.NotNil()
are used to check if the object is nil or not. It takes two arguments, the first one is the testing object and the second one is the object to be checked.
...
assert.Nil(t, nil)
assert.NotNil(t, "Hello, World!")
5. True and False
assert.True()
and assert.False()
are used to check if the object is true or false, they are both usually used with boolean values or functions returning boolean values. It takes two arguments, the first one is the testing object and the second one is the object to be checked.
...
assert.True(t, true)
assert.False(t, false)
6. Empty and NotEmpty
assert.Empty()
and assert.NotEmpty()
are used to check if the object is empty or not. It takes two arguments, the first one is the testing object and the second one is the object to be checked.
...
assert.Empty(t, "")
assert.NotEmpty(t, "Hello, World!")
7. Len
assert.Len()
is used to check if the length of the object is equal to the expected length. It takes three arguments, the first one is the testing object, the second one is the object to be checked and the third one is the expected length.
...
j := []int{1, 2, 3}
assert.Len(t, j, 3)
8. Contains
assert.Contains()
is used to check if the object contains the expected value. It takes three arguments, the first one is the testing object, the second one is the object to be checked and the third one is the expected value.
...
j := []int{1, 2, 3}
assert.Contains(t, j, 2)
9. Panics and NotPanics
assert.Panics()
and assert.NotPanics()
is used to check if the function panics or not. It takes two arguments, the first one is the testing object and the second one is the function to be checked.
...
func willPanic() {
panic("Panic!")
}
func willNotPanic() {
fmt.Println("Hello, World!")
}
func TestPanic(t *testing.T) {
assert.Panics(t, willPanic)
assert.NotPanics(t, willNotPanic)
}
10. Error and NoError
assert.Error()
and assert.NoError()
is used to check if the error is nil or not. It takes two arguments, the first one is the testing object and the second one is the error to be checked.
...
func willError() error {
return errors.New("Error!")
}
func willNotError() error {
return nil
}
func TestError(t *testing.T) {
assert.Error(t, willError())
assert.NoError(t, willNotError())
}
Conclusion
writing tests is a very important part of the development process, and it is very important to write tests for your code. In this article, we have learned how to use the assert package in Golang. We have also learned about the major assert methods. I hope you have enjoyed this article.
Extend your knowledge with these articles:
-
Writting Tests in Golang (A Complete Guide)
- Golang Benchmarking If you have any questions or suggestions, please reach out to me on Twitter.