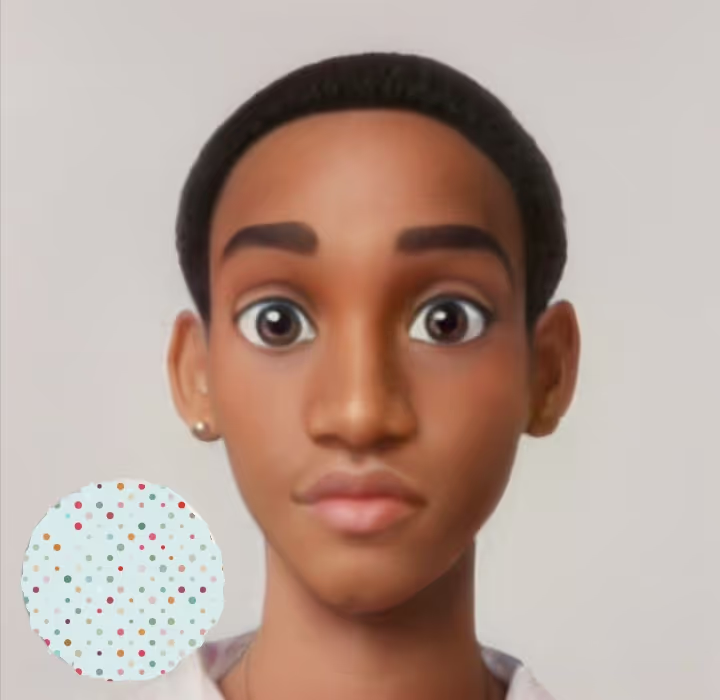
Golang Atoi
What is Atoi in Golang?
Atoi is a method in the strconv package that converts a string to an integer. It returns an error if the string cannot be converted to an integer. The strconv package is part of the standard library.
How to use Atoi in Golang
The Atoi function takes a string and returns an integer and an error. The error is nil if the string can be converted to an integer. If the string cannot be converted to an integer, the error is not nil.
package main
import (
"fmt"
"strconv"
)
func main() {
i, err := strconv.Atoi("42")
if err != nil {
fmt.Println(err)
}
fmt.Println(i)
}
In the example above, the string “42” is converted to an integer and assigned to the variable i
. The error is not nil because the string “42” can be converted to an integer. The error is nil and the integer 42 is printed to the console.
...
i, err := strconv.Atoi("forty-two")
if err != nil {
fmt.Println(err)
}
fmt.Println(i)
In the example above, the string “forty-two” is passed to the Atoi
function. The string cannot be converted to an integer and the error is not nil. The error is printed to the console and the integer 0 is printed to the console.
In Go int variables are initialized to 0 by default. If you try to convert a string to an integer and the string cannot be converted to an integer, the integer will be initialized to 0.
convert to int32 in Golang
The Atoi
function converts a string to an integer. The integer is an int type. If you need to convert a string to an int32, you can use the Atoi
function and then cast the integer to an int32.
...
i, err := strconv.Atoi("42")
if err != nil {
fmt.Println(err)
}
i32 := int32(i)
fmt.Println(i32)
The int type is a 32-bit integer on 32-bit systems and a 64-bit integer on 64-bit systems.
convert to int64 in Golang
The Atoi
function converts a string to an integer. The integer is an int type. If you need to convert a string to an int64, you can use the Atoi
function and then cast the integer to an int64.
...
i, err := strconv.Atoi("42")
if err != nil {
fmt.Println(err)
}
i64 := int64(i)
fmt.Println(i64)
convert to uint in Golang
The Atoi
function converts a string to an integer. The integer is an int type. If you need to convert a string to a uint, you can use the Atoi
function and then cast the integer to a uint.
...
i, err := strconv.Atoi("42")
if err != nil {
fmt.Println(err)
}
u := uint(i)
fmt.Println(u)
The most convenient way to do integer conversions to a particular byte size is
to use the strconv.ParseInt()
function.
Atoi vs ParseInt in Golang
The Atoi()
method is a wrapper for the ParseInt()
method. The Atoi()
method is a convenience method that calls the ParseInt()
method with the base 10 and bit size 0. The ParseInt()
method is more flexible because it allows you to specify the base and bit size.
...
i, err := strconv.Atoi("42")
if err != nil {
fmt.Println(err)
}
fmt.Println(i)
i64, err := strconv.ParseInt("42", 10, 64)
if err != nil {
fmt.Println(err)
}
fmt.Println(i64)
In the example above, the Atoi()
method is used to convert the string “42” to an integer. The ParseInt()
method is used to convert the string “42” to an integer. The Atoi()
method is a wrapper for the ParseInt()
method.