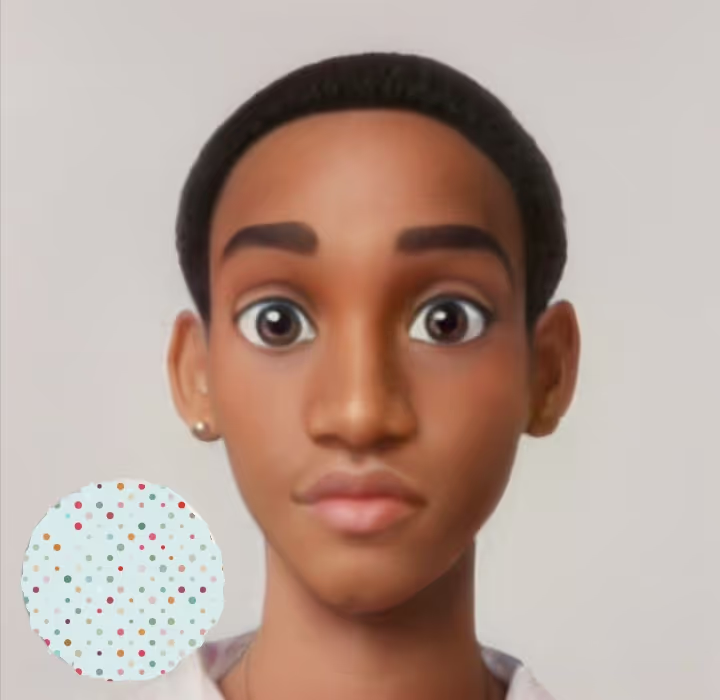
Golang constants
Introduction
In Go, a constant is an immutable value that can be used in place of a variable. Constants can be string, boolean, or numeric values. Constants cannot be declared using the :=
syntax.
How to declare a constant
Constants are declared like variables, but with the const
keyword. The :=
syntax is only used for declaring variables. This forms one of the main differences between constants and variables.
package main
import "fmt"
const Pi = 3.14
func main() {
fmt.Println(Pi)
}
Pi
is a constant of type float64
and can be used in place of a variable.
How to declare multiple constants
To declare multiple constants, use the const
and place the variables in a parenthesis.
package main
import "fmt"
func main() {
const (
Pi = 3.14
Language = "Go"
Version = 1.19
)
fmt.Println(Pi, Language, Version)
}
Here we have declared three constants of type float64
, string
, and int
.
The output of the above code is:
3.14 Go 1.19
How to declare a constant with an explicit type
Even though constants are immutable, they can still have an explicit type. This is done by placing the type after the constant name.
package main
import "fmt"
func main() {
const (
Pi float64 = 3.14
Language string = "Go"
Random int = 10e6
)
fmt.Printf("%T %T %T\n", Pi, Language, Version)
}
The output of the above code is:
float64 string int
How to declare custom types as constants
The same rules apply to custom types as they do to built-in types. This means that custom types can be declared as constants.
package main
import "fmt"
type Language string
const (
Go Language = "Go"
Python Language = "Python"
JavaScript Language = "JavaScript"
)
func main() {
fmt.Println(Go, Python, JavaScript)
}
Only custom types that are based on built-in types that can be constants can be constants.
Constant edge cases
Maps cannot be constants
Maps cannot be constants because they are mutable. This is because the length of a map is not known at compile time.
package main
import "fmt"
func main() {
const m = map[string]int{
"one": 1,
"two": 2,
}
fmt.Println(m)
}
The output of the above code is:
./main.go:6:13: map[string]int{…} (value of type map[string]int) is not constant
Constant values should be known at compile time
Constant values should be known at compile time. This means that the value of a constant should be able to be determined at compile time. This includes values that are the result of a function call.
package main
import "fmt"
func main() {
const Pi = math.Pi
fmt.Println(Pi)
}
The output of the above code is:
3.14
This is because math.Pi
is a constant and can be determined at compile time.
If we were to introduce a function call, the compiler would throw an error.
package main
import (
"fmt"
"math"
)
func main() {
const Pi = math.Sin(1.57)
fmt.Println(Pi)
}
The output of the above code is:
./expr.go:12:13: math.Sin(1.57) (value of type float64) is not constant
Function calls are not allowed in constants because the value of the function call cannot be determined at compile time.
Other types that cannot be used in constants are:
- Function calls
- Array and slice literals
Conclusion
In this article, we have learned how to declare constants in Go. We have also learned about some of the edge cases that can occur when declaring constants.