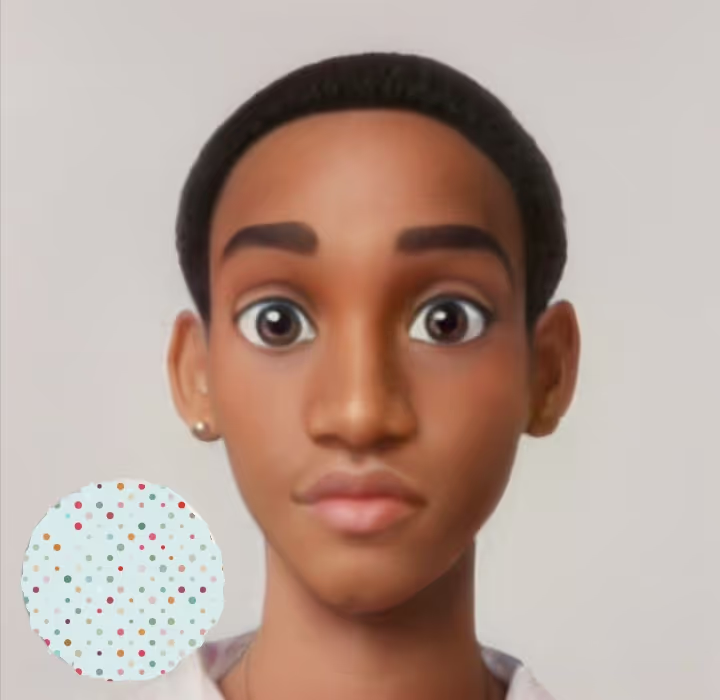
Golang If Else Statements (A Complete Guide)
Introduction
If-else statements are a fundamental part of programming, and Golang is no exception. They allow you to control the flow of your code by executing certain sections of it based on certain conditions. In this article, we will be taking a deep dive into the use of if-else statements in Golang. We will cover everything you need to know about if-else syntax, conditions, and nested statements to help you write efficient and reliable code. Whether you’re a beginner or an experienced developer, this guide will give you a comprehensive understanding of if-else statements in Golang. So, let’s get started
What is an If-Else Statement?
An if-else statement is a control flow statement that allows you to execute certain sections of code based on certain conditions. If the condition is true, the code inside the if block will be executed. If the condition is false, the code inside the else block will be executed.
If-Else Syntax
The syntax of an if-else statement in Golang is as follows:
if condition {
// code to be executed if the condition is true
} else {
// code to be executed if the condition is false
}
If-Else Conditions in Golang
The condition in an if-else statement can be any valid boolean expression. It can be a simple boolean variable, a comparison, a logical expression, or a function call that returns a boolean value.
package main
import "fmt"
func main() {
isTrue := true
if isTrue {
fmt.Println("isTrue is true")
} else {
fmt.Println("isTrue is false")
}
if 1 == 1 {
fmt.Println("1 is equal to 1")
} else {
fmt.Println("1 is not equal to 1")
}
}
Nested If-Else Statements in Golang
You can also nest if-else statements inside each other. This allows you to create complex conditions and execute different sections of code based on the result of each condition.
package main
import "fmt"
func main(){
num := 10
if num > 0 {
if num % 2 == 0 {
fmt.Println("num is a positive even number")
} else {
fmt.Println("num is a positive odd number")
}
} else {
if num % 2 == 0 {
fmt.Println("num is a negative even number")
} else {
fmt.Println("num is a negative odd number")
}
}
}
Else If Statements in Golang
You can also chain if-else statements together. This allows you to create a series of conditions that will be executed in order. If the first condition is false, the next condition will be checked, and so on. If none of the conditions are true, the code outside the if-else statement will be executed.
package main
import "fmt"
func main(){
num := 10
if num > 0 {
fmt.Println("num is a positive number")
} else if num < 0 {
fmt.Println("num is a negative number")
} else {
fmt.Println("num is zero")
}
}
Conclusion
This article has provided a comprehensive guide on if-else statements in Golang and with the examples and explanations provided, you should now have a solid understanding of how to use them effectively in your code.