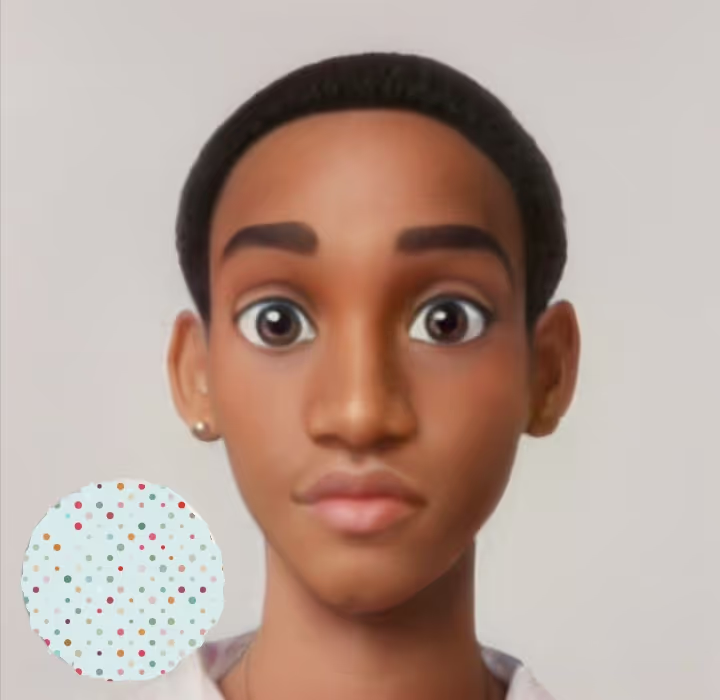
Golang Runes
What is a Rune?
A rune is a built-in data type in Golang that represents a single Unicode character and is an alias for the int32
type. Unicode is a character encoding standard that allows for the representation of all characters in all languages, unlike ASCII which only supports English characters. The int32
type is a 32-bit integer representing all Unicode characters. Unlike strings which are a sequence of bytes, runes are a sequence of characters. Since strings in Go are of UTF-8 encoding a string can hold Unicode characters by representing them as a sequence of 4 bytes.
Rune Defenition and Initialization
A rune is defined by enclosing a character in single quotes. By enclosing in a single quote, a rune literal is created.
a := 'a'
var b rune = 'b'
c := '🧯'
In this example, we have created three rune variables. The first two are initialized with a single character. The third is initialized with a Unicode character (emoji).
fmt.Printf("Value of a is %v and type is %T\n", a, a)
fmt.Printf("Value of b is %v and type is %T\n", b, b)
fmt.Printf("Value of c is %v and type is %T\n", c, c)
By printing the value and type of each rune, we can see the int32
representation of each character and the int32
type.
A rune can also be initialized with a Unicode code point. A Unicode code point is a number that represents a character in the Unicode standard. We can use the rune()
function to initialize a rune with a Unicode code point.
d := rune(0x1F9EF)
fmt.Printf("Value of d is %v and type is %T\n", d, d)
Another way is using the \u
escape sequence.
e := '\u1F9EF'
fmt.Printf("Value of e is %v and type is %T\n", e, e)
Rune to String Conversion and Vice Versa
A rune can be converted to a string using the string()
function.
f := string('a')
fmt.Println(f)
A string can be converted to a rune using the []rune()
function, which returns a slice of runes.
wr := "Runes are cool!"
fmt.Println([]rune(wr))